Create an image with three different aspect ratios
To be clear - this will create three different images by making three API calls - but using the same prompt and using different aspect rations for the resulting image.
The x,y pixel sizes are defined in the layouts
variable.
import requests, json
from IPython.display import Image
api_key ="<YOUR API KEY HERE"
layouts = [ [1024,1024], [768, 1024], [1024,768]]
prompt = "A highschool yearbook photo of a young man from the 1980s. Laser light background."
imageurls = []
url = "https://api.corcel.io/v1/image/vision/text-to-image"
for layout in layouts:
payload = {
"cfg_scale": 2,
"height": layout[0],
"width": layout[1],
"steps": 8,
"engine": "proteus",
"text_prompts": [{ "text": prompt }]}
headers = {
"accept": "application/json",
"content-type": "application/json",
"Authorization": api_key
}
response = requests.post(url, json=payload, headers=headers)
resJson = json.loads(response.text)
imageurl = resJson['signed_urls'][0]
print(imageurl)
imageurls.append(imageurl)
for image in imageurls:
display(Image(url=image))
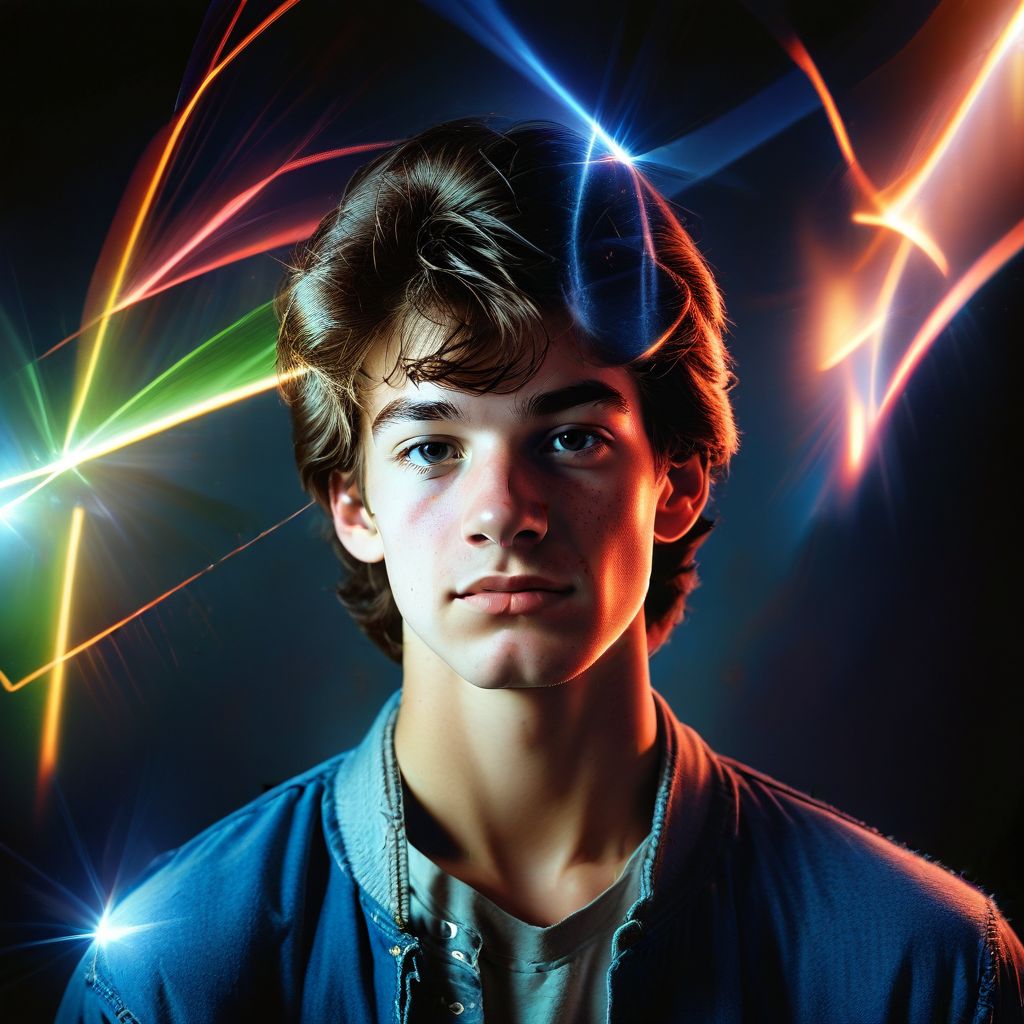
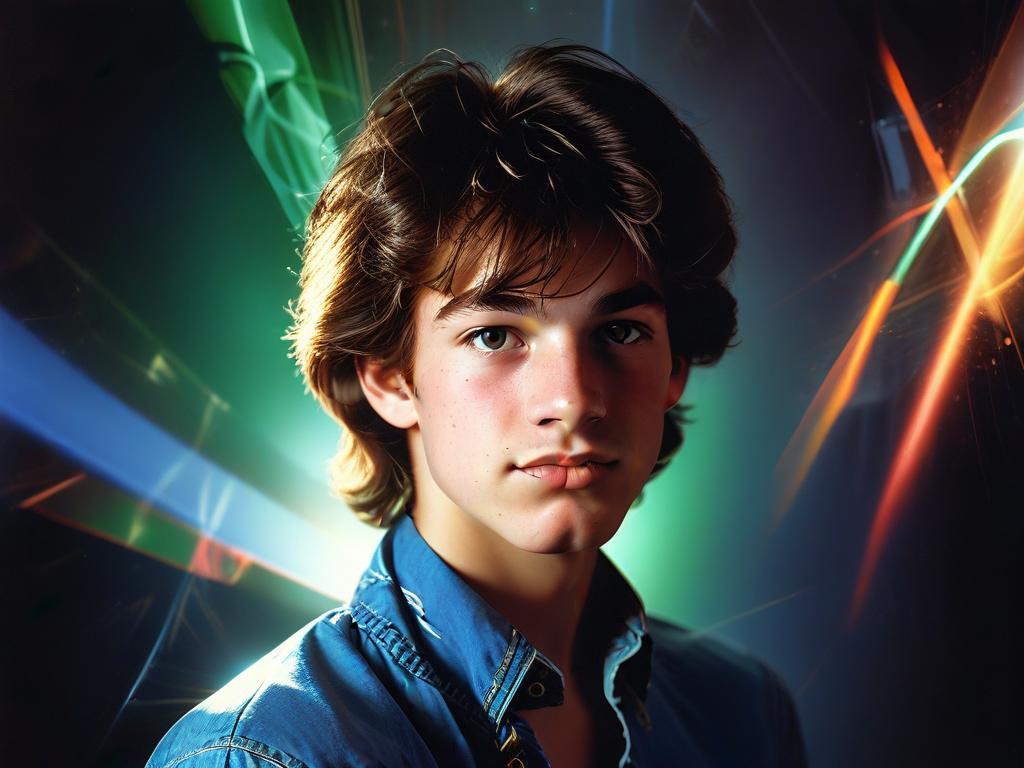
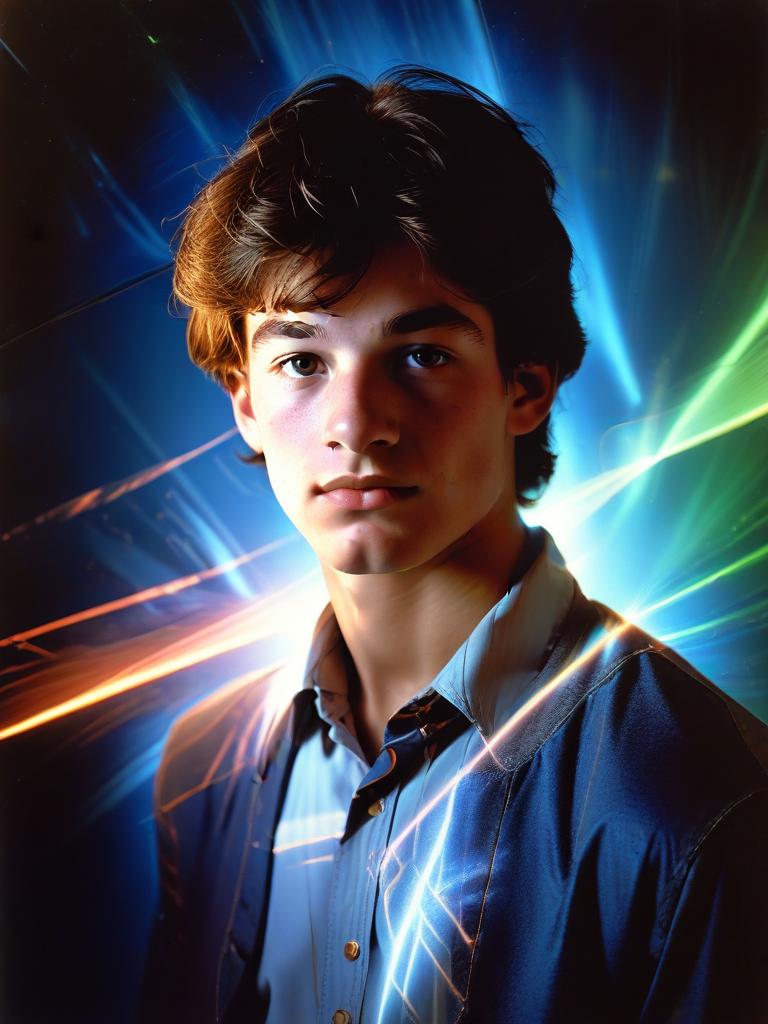
Updated 7 months ago